Splitting Strings in Swift
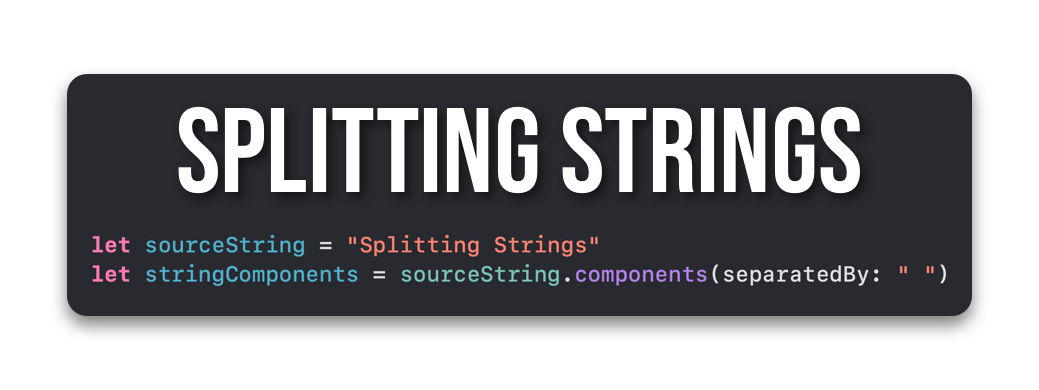
So this actually came up at work the other day and I felt like it was a good thing to write up for others in case they come across anything similar while building apps using Swift. The problem was a string sent back from the server needed to be split up into two strings - a title and a description. The title and description were separated by a special character, which made splitting the string up super easy.
Let’s take the following string as an example:
“Splitting Strings&How to do it in Swift”
We want to split the string into two components; one for the title “Splitting Strings” and one for the description “How to do it in Swift.” To do that, we’ll use components(separatedBy:)
let sourceString = "Splitting Strings&How to do it in Swift"
let stringComponents = sourceString.components(separatedBy: "&")
Now stringComponents
is an array of our two strings that can be used on their own, with the separator “&”
thrown out. Quick and easy!
Check out some other recent tutorials
Animations in your UI are a must these days, but everyone is doing the same old fade or move animations. If you really want to take your animations to the next level you need to incorporate some rotation.
Views in SwiftUI can be added to and removed from other views easily by checking a state property. When views are added or removed using just a normal bool check they are automatically given a fade in/out transition by default. That’s great for a lot of cases, but what if you want to do something different, like show a message coming in from the top?
Showing an alert is a great way to notify a user of important information related to your app such as a payment going through or an error loading something from the server. In SwiftUI Apple has added a modifier that makes it super easy to show an alert based off your view’s state.
Lists are great for displaying vertically scrolling collections of data. But what if you want something that scrolls horizontally? There isn’t a fully native SwiftUI version of UICollectionView yet, so for the time being you have to get a little creative using a combination of ScrollView and HStack.
Today we’re going to look at how to create a quick and easy animation for a button that scales and fades out when you tap on it.
Learn how to use protocols and extensions to make dequeueing cells super easy and optional free.
A best practice in SwiftUI architecture is to break views down into smaller components that you combine together to build more complex views. Learn how to do just that by building custom List rows in this tutorial!
It took me longer than I’d care to admit to figure out how to set the background color for a full screen view in SwiftUI. Read on to avoid my mistakes and learn how to quickly and easily set the background color for your views!
Being able to enter text into an app is essential. In this short tutorial you’ll learn how to create a TextField
, bind it to a value, and update its style so you can give your users a way to enter text in your apps.